BinaryTree
小于 1 分钟
二叉树
BinaryTree
- 树的深度
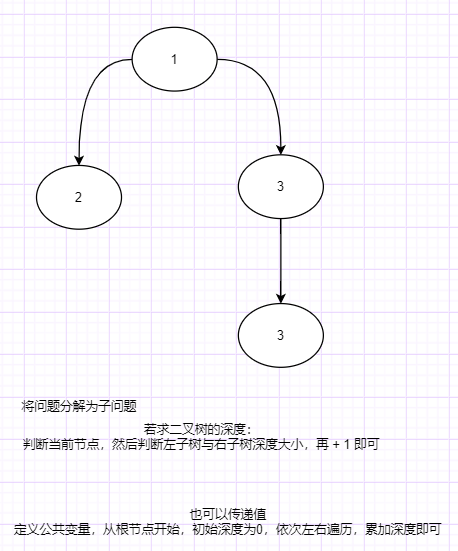
- 形状
public List<Integer> rightSideView(TreeNode root) {
List<Integer> res = new ArrayList<>();
height(root, 0, res);
return res;
}
public void height(TreeNode node, int depth, List<Integer> res) {
// 先读右子树,再读左子树,res 个数与二叉树的深度相同
if (node == null) {
return;
}
if (depth == res.size()) {
res.add(node.val);
}
height(node.right, depth + 1, res);
height(node.left, depth + 1, res);
}
找公共祖先
bfs
class Solution {
public List<List<Integer>> levelOrder(TreeNode root) {
// bfs
if (root == null) {
return List.of();
}
List<List<Integer>> res = new ArrayList<>();
Queue<TreeNode> queue = new LinkedList<>();
queue.add(root);
while(!queue.isEmpty()) {
int n = queue.size();
List<Integer> list = new LinkedList();
while(n-- > 0) {
TreeNode node = queue.poll();
list.add(node);
if (node.left != null) {
queue.add(node.left);
}
if (node.right != null) {
queue.add(node.right);
}
}
res.add(list);
}
return res;
}
}